Understanding Async and Await in C#
What is Async and Await in C#?
Async and Await are essential components of asynchronous programming in C#. They allow applications to execute tasks without blocking the main thread, enabling better responsiveness and efficient execution.
The async
keyword is used to declare an asynchronous method, while the await
keyword pauses the method execution until the awaited task completes. This prevents blocking operations and allows other tasks to continue running.
How Async and Await Work?
When an asynchronous method is called, it starts executing but returns control to the caller immediately while waiting for the awaited task to complete. This ensures non-blocking execution.
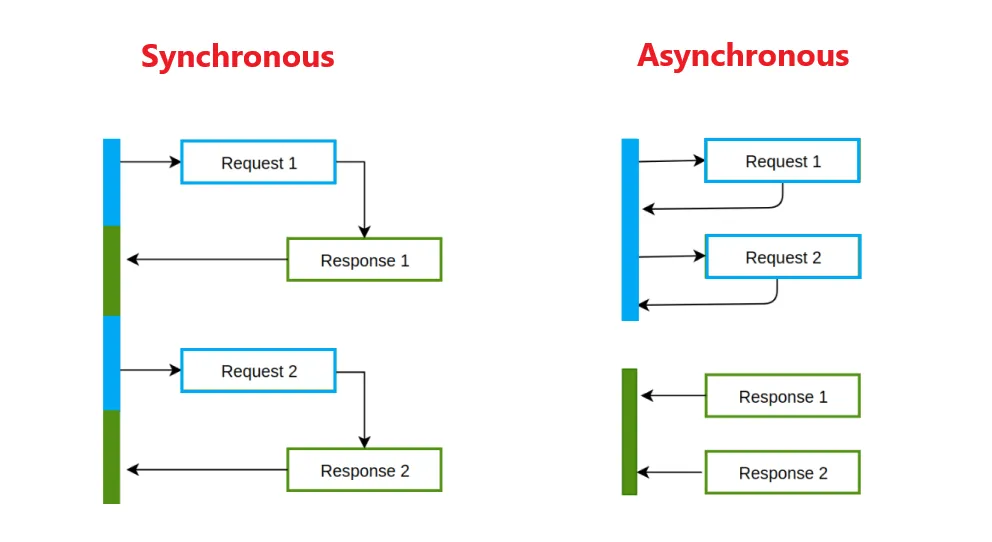
The diagram above illustrates how async methods handle tasks without blocking the main thread.
Benefits of Using Async and Await
- Improves Responsiveness: Async methods allow the main thread (UI thread in GUI apps) to remain free while waiting for operations to complete.
- Better Resource Utilization: Enables concurrent execution of tasks, improving efficiency in web services and applications.
- Code Readability and Maintainability: Compared to traditional threading models, async/await simplifies complex callback-based code.
- Automatic Context Switching: After an async task completes, execution resumes on the original thread, making UI updates easier.
Drawbacks of Using Async and Await
-
Not Suitable for CPU-bound Operations: Async methods are ideal for I/O tasks but not for heavy computation; use
Task.Run
instead. -
Potential Deadlocks: Calling
.Result
or.Wait()
on async methods can block the main thread and cause deadlocks. - Overhead for Small Tasks: If an operation is quick (e.g., simple calculations), adding async introduces unnecessary overhead.
- Complex Debugging: Call stacks in asynchronous code may be incomplete, making debugging harder compared to synchronous methods.
Best Practices for Using Async and Await
-
Always use
await
instead of.Result
or.Wait()
to prevent deadlocks. -
Use
ConfigureAwait(false)
in library code to avoid unnecessary context switching. -
Avoid making async methods
void
; useTask
orTask<T>
for proper exception handling. - Only use async where necessary—don't make everything async by default.
Async vs Sync Execution: Code Example
Synchronous Execution:
public void SyncMethod()
{
Console.WriteLine("Task started");
Thread.Sleep(3000); // Blocks the main thread
Console.WriteLine("Task completed after 3 seconds");
}
// Usage
SyncMethod();
Asynchronous Execution:
public async Task AsyncMethod()
{
Console.WriteLine("Task started");
await Task.Delay(3000); // Non-blocking delay
Console.WriteLine("Task completed after 3 seconds");
}
// Usage
await AsyncMethod();
With async execution, the main thread remains free to handle other tasks.
When to Use Async and Await?
Use Case | Recommended? |
---|---|
Web API Calls | ✅ Yes, prevents blocking requests |
Database Queries | ✅ Yes, improves efficiency |
File I/O (Read/Write) | ✅ Yes, avoids blocking disk operations |
UI Event Handlers | ✅ Yes, keeps UI responsive |
CPU-Intensive Work (Calculations) | ❌ No, use Task.Run instead |
Small, Simple Methods | ❌ No, avoid unnecessary async overhead |